기본 패키지생성
src/main/java
폴더 하위에 아래 4가지 패키지가 기본으로 있어야한다.
- controller
- domain
- persistence
- service
src/main/resources
폴더 하위에 mapper폴더를 생성한다.

DB 테이블생성
컬럼과 제약조건을 포함하여 게시판 테이블을 생성한다.
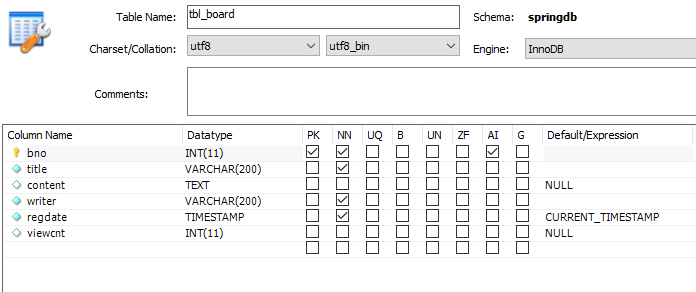
web.xml
한글처리 코드 추가
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <filter> <filter-name>encoding</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> </filter>
<filter-mapping> <filter-name>encoding</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
|
부트스트랩 템플릿 적용하기
원하는 부트 스트랩으로 뷰페이지를 생성한 뒤 컨트롤러로 연결한다
boardVO.java
도메인생성
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| public class BoardVO {
private Integer bno; private String title; private String content; private String writer; private Timestamp regdate; private int viewcnt; public BoardVO() {} public BoardVO(Integer bno, String title, String content, String writer, Timestamp regdate, int viewcnt) { super(); this.bno = bno; this.title = title; this.content = content; this.writer = writer; this.regdate = regdate; this.viewcnt = viewcnt; }
public Integer getBno() { return bno; }
public void setBno(Integer bno) { this.bno = bno; } }
|
root-context.xml
코드 추가
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context" xmlns:jdbc="http://www.springframework.org/schema/jdbc" xmlns:mybatis-spring="http://mybatis.org/schema/mybatis-spring" xsi:schemaLocation="http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-4.3.xsd http://mybatis.org/schema/mybatis-spring http://mybatis.org/schema/mybatis-spring-1.2.xsd http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd"> <bean id="datasource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="net.sf.log4jdbc.sql.jdbcapi.DriverSpy" /> <property name="url" value="jdbc:log4jdbc:mysql://localhost:3306/springdb?useSSL=false"/> <property name="username"> <value>root</value> </property> <property name="password"> <value>1234</value> </property> </bean> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <property name="dataSource" ref="dataSource" /> <property name="configLocation" value="classpath:/Mybatis-config.xml" /> <property name="mapperLocations" value="classpath:mappers/**/*Mapper.xml" /> </bean> <bean id="sqlSession" class="org.mybatis.spring.SqlSessionTemplate" destroy-method="clearCache"> <constructor-arg name="sqlSessionFactory" ref="sqlSessionFactory" /> </bean> <context:component-scan base-package="com.itwillbs.persistence" /> <context:component-scan base-package="com.itwillbs.service" /> </beans>
|
BoardDAO.java
인터페이스에 메서드 추가
1 2 3 4 5 6 7 8
| package com.itwillbs.persistence;
import com.itwillbs.domain.BoardVO;
public interface BoardDAO { public void create(BoardVO vo) throws Exception; }
|
BoardDAOImpl.java
메서드 오버라이딩 코드 추가
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| package com.itwillbs.persistence;
import javax.inject.Inject; import org.apache.ibatis.session.SqlSession; import com.itwillbs.domain.BoardVO;
public class BoardDAOImpl implements BoardDAO {
@Inject private SqlSession session; private static final String namespace = "com.itwillbs.mapper.BoardMapper"; @Override public void create(BoardVO vo) throws Exception { session.insert(namespace+".create", vo); System.out.println("DAO 글쓰기메서드"); } }
|
boardMapper.xml
SQL쿼리 추가
- mapper 명 작성하는 룰은 고정이다. =>
도메인명Mapper.xml
1 2 3 4 5 6 7 8 9 10 11 12
| <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.itwillbs.mapper.BoardMapper"> <insert id="create"> insert into tbl_board (title,content,writer) values (#{title},#{content},#{writer}) </insert> </mapper>
|
테스트 파일 BoardDAOTest.java
생성
- src/test/java 하위에 test 파일인
BoardDAOTest.java
를 생성한다.
- 서비스는 컨트롤러 역할의 테스트 객체이다.
- 아래 코드를 작성하고 서버를 run하면 콘솔과 DB에 데이터를 확인할 수 있다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| package com.itwiilbs.controller;
import javax.inject.Inject;
import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import com.itwillbs.domain.BoardVO; import com.itwillbs.persistence.BoardDAO;
@RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = {"file:src/main/webapp/WEB-INF/spring/root-context.xml"}) public class BoardDAOTest { @Inject private BoardDAO bdao; @Test public void testDAO() throws Exception{ System.out.println("TEST: boardDAO -> bdao"); } @Test public void testInsertBoard() throws Exception{ BoardVO vo = new BoardVO(); vo.setTitle("공지사항"); vo.setContent("공지안내입니다. 테스트입니다."); vo.setWriter("관리자"); bdao.create(vo); System.out.println("T: 글쓰기메서드 "+vo); } }
|