문제 1018 : [기초-입출력] 시간 입력받아 그대로 출력하기
시(hour)와 분(minute)이 “:” 으로 구분된 형식에 맞추어 시간이 입력될 때, 그대로 출력하는 연습을 해보자.
코드1 : nextLine()이용
1 2 3 4 5 6 7 8 9 10
| import java.util.Scanner;
class Main { public static void main(String[] args) { Scanner scan = new Scanner(System.in); String time = scan.nextLine(); scan.close(); System.out.printf("%s", time); } }
|
코드2 : Integer.parseInt()이용
1 2 3 4 5 6 7 8 9 10 11 12
| import java.util.Scanner;
class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); String str = sc.next(); sc.close();
String[] time = str.split(":"); System.out.printf("%d:%d", Integer.parseInt(time[0]), Integer.parseInt(time[1])); } }
|
코드3 : Integer.valueOf()이용
1 2 3 4 5 6 7 8 9 10 11 12
| import java.util.Scanner;
class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); String str = sc.next(); String[] time = str.split(":"); sc.close();
System.out.printf("%d:%d", Integer.valueOf(time[0]),Integer.valueOf(time[1])); } }
|
세 코드 비교
Scanner를 close 유무로 수행시간과 메모리 차이가 발생한다.
|
nextLine() |
Integer.parseInt() |
Integer.valueOf() |
close 전 수행시간 |
109ms |
110ms |
109ms |
close 후 수행시간 |
109ms |
114ms |
113ms |
close 전 메모리 |
14960kb |
15028kb |
15004kb |
close 후 메모리 |
14972kb |
14976kb |
14972kb |
next()와 nextLine()차이
- next() : 공백을 기준으로 입력.
- nextLine() : 한 라인을 기준으로 입력.
1 2 3 4 5 6 7 8 9 10 11 12 13
|
String n = sc.next(); System.out.println(n);
String nl = sc.nextLine(); System.out.println(nl);
가나다 가나다 라 마 바 사
|
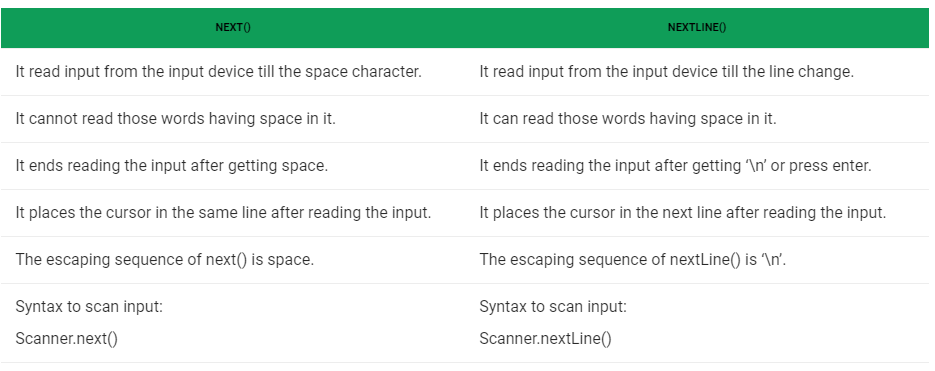
parseInt, valueOf의 차이
다른 문제 풀이가 보고싶다면?